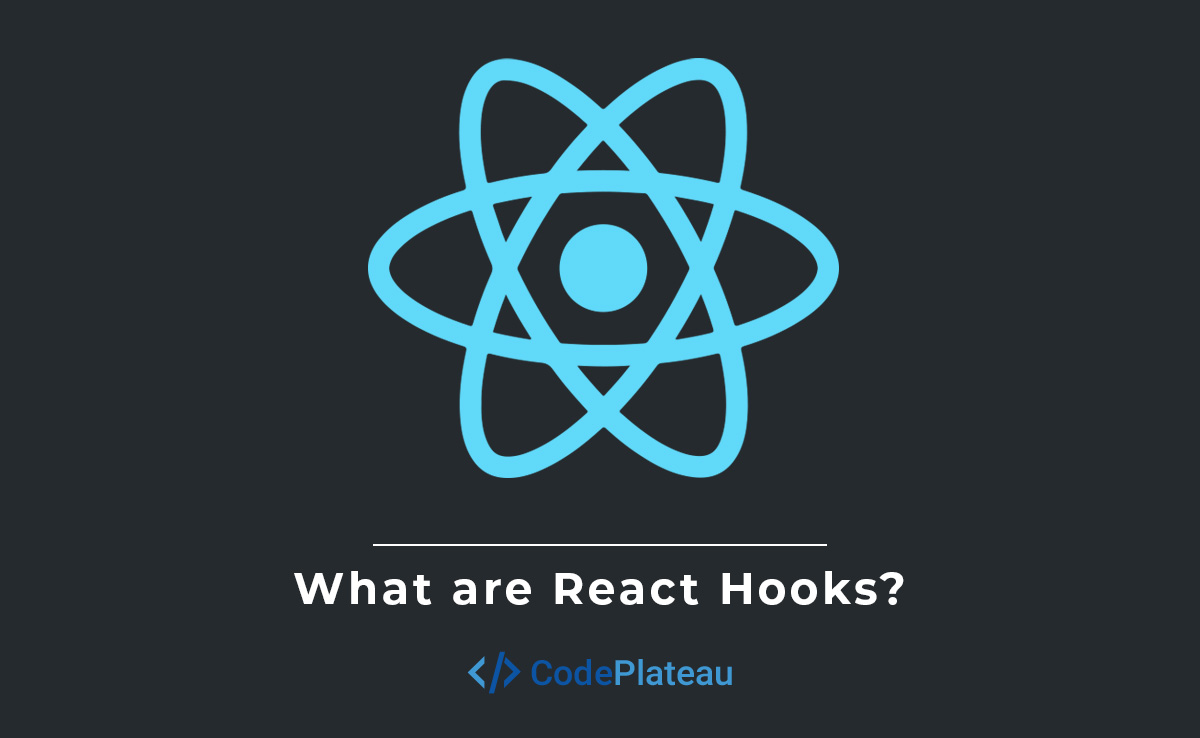
React Hooks were introduced at React Conf October 2018, where two major functional
components were highlighted – useState and useEffect. Function compontents were initially known as functional stateless components (FSC), where they are finally able to
useState with React Hooks. Therefore, many people refer them as function
components.
Hooks let you “hook into” the underlying lifecycle and state changes of a component
within a functional component. More than that, they often also improve readability and
organization of your components. We can check 2 hooks that are used regularly while
using React Js.
1) useState:
import React from 'react';
function App() {
return (
<div>
<h1>0</h1>
<button>Change!</button>
</div>
);
}
In this above example, this is a simple functional component in which we can import our
first hooks useState for Handel state data.
import React, { useState } from 'react';
function App() {
const value = useState();
console.log(value);
return (
<div>
<h1>0</h1>
<button>Change!</button>
</div>
);}
If we run this code and console the data then we can get a response as below
> [null, ƒ()]
And if we add an argument into use state
const value = useState(true);
we can get a response as below
> [true, ƒ()]
Now we can access state value and render it in <h1> in our component such as
import React, { useState } from 'react';
function App() {
const value = useState(0);
console.log(value); // [0, ƒ()]
return (
<div>
<h1>{value[0]}</h1>
<button>Change!</button>
</div>
);
}
There are 2 types of functionality that store data into use state
1) Object destructuring
2) Array destructuring
Array destructing is almost the same, but uses square brackets [ ] instead of curly
braces { }.
Using array destructuring, we can get the initial value of state from the useState() hook.
import React, { useState } from 'react';
function App() {
// remember, there's a second item from the array that's missing here, but we'll come
right back to use it soon
const [count] = useState(0);
return (
<div>
<h1>{count}</h1>
<button>Change!</button>
</div>
);
}
Right now we can get initial state value but how we can change the value in hooks that
are described in the below example
function App() {
const [count, setCount] = useState(0);
function change() {
setCount(prevCount => prevCount + 1);
}
return (
<div>
<h1>{count}</h1>
<button onClick={change}>Change!</button>
</div>
);
}
Here we first set initial count to use state is 0 then for an update that
counts we can use onclick event listener on button click.
Remember that useState() hook returns an array with 2 members. The second
member is a function that updates the state!
2)useEffect
In class-based components, we needed to know the basics of lifecycle methods and which
method is perfect for different situations. useEffect hook simplified this situation. If you wish to
perform side effects, network request, manual DOM manipulation, event listeners or timeouts
and intervals.
useEffect hook can be imported just like useState.
import React, { useState, useEffect } from 'react';
To make useEffect work, we pass it an anonymous function as an argument. Whenever React
re-renders this component, it will run the function we pass to useEffect
useEffect will run every time the component re-renders, and the component will re-render every
time the state is changed.
So if we write the following code, it will get us stuck in an infinite loop! This is a very common
gotcha with useEffect
If you want to call useEffect on some call function that also possible in react hooks.
No comment yet, add your voice below!